An effector is executed when its transition is fired. An effector can have an effect upon the various contexts of the simulation. Transitions can usually hold any number of effectors.
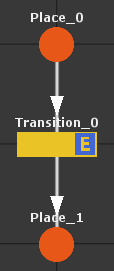
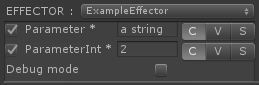
A transition with an effector between two places.
To create a Unity effector; create a new C# script within the project. For convenience, the script should end up by Effector. It should be located within an Effectors folder.

How to create an effector for Unity?
A Unity effector must inherit from AUnityEffector. It helps building an effector that will execute code within the Unity thread. An effector that does not require some code to run within an Unity thread can directly inherit from AEffector.
You need to call the base constructor when inheriting this class and implement the Init() and SafeEffectorUpdate() methods.
- SafeReset() is responsible of the effector initialization. It will be called in Unity's thread after the field and properties values are set and everytime the effector must be used (i.e. if the transition holding it is fired again)
- SafeEffectorUpdate() is the actual effector code that will be executed in Unity's thread, whether the scenario is threaded or not.
An effector can access various contexts given to the constructor: external context, scenario context, sequence context, event context.
- The external context corresponds to the world context created in Unity.
- The scenario context is a set of data common to the whole scenario. It can be interpreted as global variables for the scenario.
- The sequence context (or token) is a set of data contained by the token moving through the transition holding this effector. Think of it as a local variables
- The event context allows for the transmission of data between the sensor and the effector of a given transition.
An effector can contain fields and properties that can be tagged using a Configuration Parameter attribute.
You can tag a field or property with the Context Variable Attribute if the effector needs to change the value of any context. In such case, it is advised to use the Context Variable generic class for the field or property.
At runtime, after the effector is constructed, the values of the fields and properties will be filled before SafeReset is called.
using Xareus.Scenarios.Context;
using Xareus.Scenarios.Utilities;
using Xareus.Scenarios.Variables;
using Xareus.Scenarios.Unity;
[FunctionDescription("A Unity Effector")]
public class ExampleEffector : AUnityEffector
{
[ConfigurationParameter("Parameter", Necessity.Required)]
string parameter;
[ConfigurationParameter("ParameterInt", Necessity.Required)]
int parameterInt;
/// <summary>
/// The result of the operation
/// </summary>
[ContextVariable("result", "The result of the operation")]
protected ContextVariable<float> result;
public ExampleEffector(Xareus.Scenarios.Event @event,
Dictionary<string, List<string>> nameValueListMap,
IContext externalContext,
IContext scenarioContext,
IContext sequenceContext,
IContext eventContext)
: base(@event, nameValueListMap, externalContext, scenarioContext, sequenceContext, eventContext)
{ }
public override void SafeReset()
{
//Initial operations
}
public override void SafeEffectorUpdate()
{
//Actions to do once the transition is fired
result.Set(5f);
}
}
Note
Several effectors are shipped with Xareus, some of them are open sourced so you can use them as an inspiration for your custom effectors.